I searched for a well done ISO countrycode table SQL script with German name attribute but couldn’t find any. So I decided to create my own. You can find it in the following GitHub repository:
SQL Scripts GitHub Repository with ISO countrycodes
JSON Viewer extension for Visual Studio
While working on a client project I needed a tool to easily compare Json files with. After a short google search I found an extension for Visual Studio called JSON Viewer. I installed the extension and it’s a pretty nice tool for formatting and comparing Json files.
By the way: If you just want to format your Json file you don’t need any additional tool because pressing Ctrl + K + D is enough to fromat your Json file.
You can find the visual studio marketplace site for the extension here: https://marketplace.visualstudio.com/items?itemName=MykolaTarasyuk.JSONViewer
Missing compiler required member ‚Microsoft.CSharp.RuntimeBinder.CSharpArgumentInfo.Create‘
You are working in a .net Core or .net Standard codebase and get the error „Missing compiler required member ‚Microsoft.CSharp.RuntimeBinder.CSharpArgumentInfo.Create“. In my case it appears when I used the dynamic type. The solution is to load the nuget package Microsoft.CSharp. After you installed it and write a using to your code everything should work as expected.
SPFx Webpart – Gulp error | Primordials is not defined
If you get the error primordials is not defined while calling a gulp command in command line the following might be the solution.
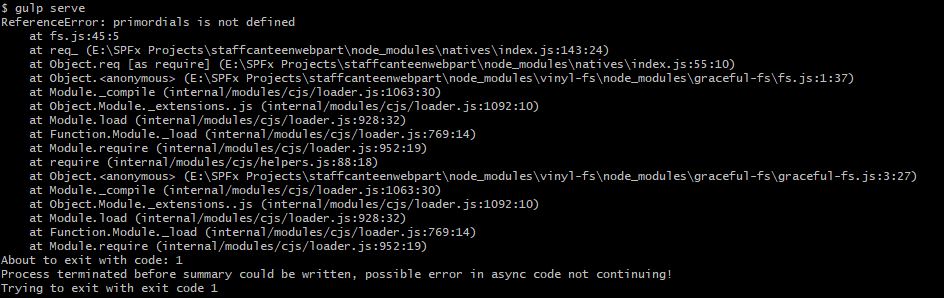
Go to your package.json file and in the node resolutions add an element graceful-fs with the LTS version in my case it was ^4.2.4. If you have Yarn installed you can now call yarn install and the dependencies are updated. When you now run the gulp command again the error could be gone.
NLog troubleshooting in Asp.Net Core
I developed a Asp.Net Core Web API application running on a 1&1 IONOS managed hosting server. For logging issues I use NLog with database target. On my local system everything worked fine and I decided to deploy it to the webserver. I called an endpoint where I excepted a log entry but nothing happened.
- Problem:
Normally you can track error issues in NLog by crating a local log file. It’s configured in the nlog.config file. On a managed system you have to get the complete directory path to set it up in the config file. You can do this by injecting IHostingEnvironment in a controller and create an action GetRootPath with no parameters. In the method you enter the following code:<br>string rootPath = _hostingEnvironment.ContentRootPath;<br>return Ok(rootPath);<br>
Now you know the root path of your application and you can set it in the nlog.config file to create a local internal log file. - Problem:
In my case I got an error like: Conversion failed when converting date and/or time from character string.
The problem was the date format for the column Logged in my NLog logging table. In my nlog.config file I set it up with<br><parameter name="@Logged" layout="${date:format=dd/MM/yyyy HH\:mm\:ss.fff}" /><br>
but the managed hosted SQL erver required a different date format and I solved the problem by set the format like<br><parameter name="@Logged" layout="${date:format=yyyy-MM-dd HH\:mm\:ss.fff}" /><br>
Sharepoint AddIn: Read items from a list by code
I started developing a Sharepoint Provider Hosted AddIn a few days ago. In this AddIn I want to read items from a list and display them in a special way. I found nearly all needed information on the Microsoft Docs. But one important information isn’t mentioned or I didn’t get it: You have to give permissions to the app otherwise you can read the list title but any item.
For giving the app permissions go to the AppManifest.xml and add a area List with the permission FullControl.
Build your project again and start it. You’ll see a Sharepoint page where you are asked if you trust your app and where you can define in a dropdown which list should be fully controlled by the app. Afterwords your app will get all items of the list if needed.
Error 405 – Methods not allowed in Asp.Net Core application
I developed an Api in Asp.Net Core Web Api. While testing it on my local machine verything works fine. When I deployed it to my Ionos Managed Windows Hosting I got a 405 Http Error on some Endpoints. Analyzing this behaviour showed me that only controller methods with PUT and DELETE annotations throwed the error.
The problem seems to be the WebDav Module of IIS blocking PUT and DELETE requests by default.
Solution: Remove WebDav in the web.config file.
<system.webServer> <handlers> <remove name="WebDAV" /> <add name="aspNetCore" path="*" verb="*" modules="AspNetCoreModuleV2" resourceType="Unspecified" /> </handlers> <modules> <remove name="WebDAVModule" /> </modules> </system.webServer>
Using Refit with Factory and Proxy
Sometimes a webhost wants you to use a proxy to communicate via http with an API for example. If you use Refit in C# with Factory implementation you can use the following code:
services.AddRefitClient() .ConfigureHttpClient(c => c.BaseAddress = new Uri(Configuration.GetValue("The name of the value in your appsettings.json"))) .ConfigurePrimaryHttpMessageHandler(() => new HttpClientHandler() { UseProxy = true, Proxy = new WebProxy("http://yourProxyUrl:yourProxyPort", false), PreAuthenticate = true, UseDefaultCredentials = false });
Set multiple startup projects in Visual Studio
There are use-cases where you want to start multiple projects of your solution at once in Visual Studio. For example if you’re developing a website in Asp.Net Core MVC getting it’s data of an Asp.Net Core Web API application. You’ll have to do the following steps to set multiple startup projects:
1) Right click on the top node of your solution in the solution explorer.
2) In the context menu choose Properties (Alt + Enter).
3) Expand the „Common Properties“ node, and choose Startup Project.
4) Select the Multiple Startup Projects radio button option and set the appropriate actions.
The way with less clicks is to click on the little arrow on the left side of the start button and choose Define Start Projects or right click on the solution node and choose Define Start Projects.
Automapper returns empty Collection after mapping
Some days ago I had a problem while mapping domain objects to DTO by automapper. My environment was an Asp.Net Core 3 Web Api project. I created an own profiles class where I stored my mapping logic. In the startup class I registered the mapper service. When running project my source of type List<Product> should be mapped to List<ProductDTO>. But the API always returned an empty list even if the source was filled correctly.
Profile:
CreateMap<Product, ProductDTO>(); CreateMap<List<Product>, List<ProductDTO>>();
The mistake was the second line in my profile class. Automapper automatically maps collections you don’t have to create a map for this case.